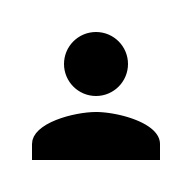
C言語でビットコインとイーサリアムで利用する暗号学的ハッシュ関数を教えて!
こういった悩みにお答えします.
本記事の信頼性
- リアルタイムシステムの研究歴12年.
- 東大教員の時に,英語でOS(Linuxカーネル)の授業.
- 2012年9月~2013年8月にアメリカのノースカロライナ大学チャペルヒル校(UNC)コンピュータサイエンス学部で客員研究員として勤務.C言語でリアルタイムLinuxの研究開発.
- プログラミング歴15年以上,習得している言語: C/C++,Python,Solidity/Vyper,Java,Ruby,Go,Rust,D,HTML/CSS/JS/PHP,MATLAB,Verse(UEFN), Assembler (x64,aarch64).
- 東大教員の時に,C++言語で開発した「LLVMコンパイラの拡張」,C言語で開発した独自のリアルタイムOS「Mcube Kernel」をGitHubにオープンソースとして公開.
- 2020年1月~現在はアメリカのノースカロライナ州チャペルヒルにあるGuarantee Happiness LLCのCTOとしてECサイト開発やWeb/SNSマーケティングの業務.2022年6月~現在はアメリカのノースカロライナ州チャペルヒルにあるJapanese Tar Heel, Inc.のCEO兼CTO.
- 最近は自然言語処理AIとイーサリアムに関する有益な情報発信に従事.
- (AI全般を含む)自然言語処理AIの論文の日本語訳や,AIチャットボット(ChatGPT,Auto-GPT,Gemini(旧Bard)など)の記事を50本以上執筆.アメリカのサンフランシスコ(広義のシリコンバレー)の会社でプロンプトエンジニア・マネージャー・Quality Assurance(QA)の業務委託の経験あり.
- (スマートコントラクトのプログラミングを含む)イーサリアムや仮想通貨全般の記事を200本以上執筆.イギリスのロンドンの会社で仮想通貨の英語の記事を日本語に翻訳する業務委託の経験あり.
こういった私から学べます.
C言語を独学で習得することは難しいです.
私にC言語の無料相談をしたいあなたは,公式LINE「ChishiroのC言語」の友だち追加をお願い致します.
私のキャパシティもあり,一定数に達したら終了しますので,今すぐ追加しましょう!
独学が難しいあなたは,元東大教員がおすすめするC言語を学べるオンラインプログラミングスクール5社で自分に合うスクールを見つけましょう.後悔はさせません!
目次
ビットコインとイーサリアムで利用する暗号学的ハッシュ関数
ビットコインとイーサリアムで利用する暗号学的ハッシュ関数を紹介します.
具体的には,SHA-2とSHA-3/Keccakを解説します.
SHA-2(SHA-256)【ビットコインで利用】
SHA-2は,SHA-1を改良版した暗号学的ハッシュ関数です.
※SHA-1はSHA-0の脆弱性を改良した暗号学的ハッシュ関数です.
SHA-2の出力長が256ビットのものとして,SHA-256があります.
ビットコインは,SHA-256を利用しています.
ビットコインを知りたいあなたはこちらからどうぞ.
SHA-3/Keccak(SHA3-256/Keccak-256)【イーサリアムで利用】
SHA-3/Keccakは,SHA-2の代替として開発された暗号学的ハッシュ関数です.
SHA-3/Keccakの出力長が256ビットのものとして,SHA3-256とKeccak-256があります.
SHA3-256とKeccak-256の違いは,後処理でのパディングルールのみです.
SHA3-256とKeccak-256の前処理と本処理は同じアルゴリズムになります.
本処理が終わった後に実行する後処理でパディングする処理が異なります.
C言語では,SHA3-256とKeccak-256の違いを,たった一行で表現できます.
イーサリアムでは,Keccak-256を利用しています.
イーサリアムを知りたいあなたはこちらからどうぞ.
SHA2(SHA-256)のコード
SHA2(SHA-256)のコードは以下になります.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 |
/* * Author: Hiroyuki Chishiro * License: 2-Clause BSD */ #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #define SHA2_HASH_SIZE 32 #define SHA2_CHUNK_SIZE 64 struct sha2 { uint8_t *hash; uint8_t chunk[SHA2_CHUNK_SIZE]; uint8_t *chunk_pos; size_t space_left; size_t total_len; uint32_t h[8]; }; #define TOTAL_LEN 8 #define BUFSIZE 128 static const uint32_t k[] = { 0x428a2f98, 0x71374491, 0xb5c0fbcf, 0xe9b5dba5, 0x3956c25b, 0x59f111f1, 0x923f82a4, 0xab1c5ed5, 0xd807aa98, 0x12835b01, 0x243185be, 0x550c7dc3, 0x72be5d74, 0x80deb1fe, 0x9bdc06a7, 0xc19bf174, 0xe49b69c1, 0xefbe4786, 0x0fc19dc6, 0x240ca1cc, 0x2de92c6f, 0x4a7484aa, 0x5cb0a9dc, 0x76f988da, 0x983e5152, 0xa831c66d, 0xb00327c8, 0xbf597fc7, 0xc6e00bf3, 0xd5a79147, 0x06ca6351, 0x14292967, 0x27b70a85, 0x2e1b2138, 0x4d2c6dfc, 0x53380d13, 0x650a7354, 0x766a0abb, 0x81c2c92e, 0x92722c85, 0xa2bfe8a1, 0xa81a664b, 0xc24b8b70, 0xc76c51a3, 0xd192e819, 0xd6990624, 0xf40e3585, 0x106aa070, 0x19a4c116, 0x1e376c08, 0x2748774c, 0x34b0bcb5, 0x391c0cb3, 0x4ed8aa4a, 0x5b9cca4f, 0x682e6ff3, 0x748f82ee, 0x78a5636f, 0x84c87814, 0x8cc70208, 0x90befffa, 0xa4506ceb, 0xbef9a3f7, 0xc67178f2 }; uint32_t right_rot(uint32_t value, unsigned int count) { return (value >> count) | (value << (32 - count)); } void consume_chunk(uint32_t *h, const uint8_t *p) { int i, j; uint32_t ah[8]; uint32_t w[16]; uint32_t s0, s1, ch, tmp, tmp2, maj; for (i = 0; i < 8; i++) { ah[i] = h[i]; } for (i = 0; i < 4; i++) { for (j = 0; j < 16; j++) { if (i == 0) { w[j] = ((uint32_t) p[0] << 24) | ((uint32_t) p[1] << 16) | ((uint32_t) p[2] << 8) | ((uint32_t) p[3]); p += 4; } else { s0 = right_rot(w[(j + 1) & 0xf], 7) ^ right_rot(w[(j + 1) & 0xf], 18) ^ (w[(j + 1) & 0xf] >> 3); s1 = right_rot(w[(j + 14) & 0xf], 17) ^ right_rot(w[(j + 14) & 0xf], 19) ^ (w[(j + 14) & 0xf] >> 10); w[j] = w[j] + s0 + w[(j + 9) & 0xf] + s1; } s1 = right_rot(ah[4], 6) ^ right_rot(ah[4], 11) ^ right_rot(ah[4], 25); ch = (ah[4] & ah[5]) ^ (~ah[4] & ah[6]); tmp = ah[7] + s1 + ch + k[i << 4 | j] + w[j]; s0 = right_rot(ah[0], 2) ^ right_rot(ah[0], 13) ^ right_rot(ah[0], 22); maj = (ah[0] & ah[1]) ^ (ah[0] & ah[2]) ^ (ah[1] & ah[2]); tmp2 = s0 + maj; ah[7] = ah[6]; ah[6] = ah[5]; ah[5] = ah[4]; ah[4] = ah[3] + tmp; ah[3] = ah[2]; ah[2] = ah[1]; ah[1] = ah[0]; ah[0] = tmp + tmp2; } } for (i = 0; i < 8; i++) { h[i] += ah[i]; } } void sha2_init(struct sha2 *sha2, uint8_t hash[SHA2_HASH_SIZE]) { sha2->hash = hash; sha2->chunk_pos = sha2->chunk; sha2->space_left = SHA2_CHUNK_SIZE; sha2->total_len = 0; sha2->h[0] = 0x6a09e667; sha2->h[1] = 0xbb67ae85; sha2->h[2] = 0x3c6ef372; sha2->h[3] = 0xa54ff53a; sha2->h[4] = 0x510e527f; sha2->h[5] = 0x9b05688c; sha2->h[6] = 0x1f83d9ab; sha2->h[7] = 0x5be0cd19; } void sha2_write(struct sha2 *sha2, const void *data, size_t len) { size_t consumed_len; const uint8_t *p = data; sha2->total_len += len; while (len > 0) { if (sha2->space_left == SHA2_CHUNK_SIZE && len >= SHA2_CHUNK_SIZE) { consume_chunk(sha2->h, p); len -= SHA2_CHUNK_SIZE; p += SHA2_CHUNK_SIZE; continue; } consumed_len = len < sha2->space_left ? len : sha2->space_left; memcpy(sha2->chunk_pos, p, consumed_len); sha2->space_left -= consumed_len; len -= consumed_len; p += consumed_len; if (sha2->space_left == 0) { consume_chunk(sha2->h, sha2->chunk); sha2->chunk_pos = sha2->chunk; sha2->space_left = SHA2_CHUNK_SIZE; } else { sha2->chunk_pos += consumed_len; } } } uint8_t *sha2_exit(struct sha2 *sha2) { uint8_t *pos = sha2->chunk_pos; size_t space_left = sha2->space_left; uint32_t *const h = sha2->h; size_t left; size_t len; int i, j; uint8_t *hash; *pos++ = 0x80; space_left--; if (space_left < TOTAL_LEN) { memset(pos, 0x00, space_left); consume_chunk(h, sha2->chunk); pos = sha2->chunk; space_left = SHA2_CHUNK_SIZE; } left = space_left - TOTAL_LEN; memset(pos, 0x00, left); pos += left; len = sha2->total_len; pos[7] = (uint8_t)(len << 3); len >>= 5; for (i = 6; i >= 0; i--) { pos[i] = (uint8_t)len; len >>= 8; } consume_chunk(h, sha2->chunk); hash = sha2->hash; for (i = 0, j = 0; i < 8; i++) { hash[j++] = (uint8_t)(h[i] >> 24); hash[j++] = (uint8_t)(h[i] >> 16); hash[j++] = (uint8_t)(h[i] >> 8); hash[j++] = (uint8_t)h[i]; } return sha2->hash; } void hash_to_string(char string[], const uint8_t hash[SHA2_HASH_SIZE]) { size_t i; for (i = 0; i < SHA2_HASH_SIZE; i++) { string += sprintf(string, "%02x", hash[i]); } } void do_sha2(char hash_string[], const char input[]) { uint8_t hash[SHA2_HASH_SIZE]; struct sha2 sha2; size_t len = strlen(input); sha2_init(&sha2, hash); sha2_write(&sha2, input, len); sha2_exit(&sha2); hash_to_string(hash_string, hash); } int main(void) { char buf[BUFSIZE]; char hash_string[BUFSIZE]; size_t len; printf("Please input a string: "); fgets(buf, SHA2_HASH_SIZE, stdin); len = strlen(buf) - 1; buf[len] = '\0'; printf("buf = %s\n", buf); do_sha2(hash_string, buf); printf("SHA-256: %s\n", hash_string); return 0; } |
空の文字列を入力した場合の実行結果は以下になります.
こちらのツールで同じ結果「e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855」になることを確認しましょう.
1 2 3 4 5 |
$ gcc sha2.c $ a.out Please input a string: buf = SHA-256: e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855 |
SHA3-256とKeccak-256のコード
SHA3-256とKeccak-256のコードは以下になります.
SHA3-256とKeccak-256の前処理と後処理は同様の結果になります.
SHA3-256とKeccak-256の後処理の違いは,以下になります.
- SHA3-256:192行目「t = (uint64_t)(((uint64_t)(0x02 | (1 << 2))) << ((ctx->byte_index) * 8));」
- Keccak-256:190行目「t = (uint64_t)(((uint64_t) 0x01) << (ctx->byte_index * 8));」
このように,C言語では,たった1行で違いを表現できていることがわかります.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 |
/* * Author: Hiroyuki Chishiro * License: 2-Clause BSD */ #include <stdio.h> #include <stdlib.h> #include <stdint.h> #include <string.h> #define BUFSIZE 128 #define SHA3_HASH_SIZE 32 #define SHA3_KECCAK_SPONGE_WORDS ((1600 / 8) / sizeof(uint64_t)) struct sha3 { uint64_t saved; union { uint64_t s[SHA3_KECCAK_SPONGE_WORDS]; uint8_t sb[SHA3_KECCAK_SPONGE_WORDS * (sizeof(uint64_t) / sizeof(uint8_t))]; } u; uint32_t byte_index; uint32_t word_index; uint32_t capacity_words; }; enum sha3_flags { SHA3_FLAGS_NONE = 0, SHA3_FLAGS_KECCAK = 1 }; #define SHA3_USE_KECCAK_FLAG 0x80000000 #define SHA3_CW(x) ((x) & (~SHA3_USE_KECCAK_FLAG)) #define SHA3_ROTL64(x, y) (((x) << (y)) | ((x) >> ((sizeof(uint64_t) * 8) - (y)))) #define KECCAK_ROUNDS 24 static const uint64_t keccakf_rndc[KECCAK_ROUNDS] = { 0x0000000000000001UL, 0x0000000000008082UL, 0x800000000000808aUL, 0x8000000080008000UL, 0x000000000000808bUL, 0x0000000080000001UL, 0x8000000080008081UL, 0x8000000000008009UL, 0x000000000000008aUL, 0x0000000000000088UL, 0x0000000080008009UL, 0x000000008000000aUL, 0x000000008000808bUL, 0x800000000000008bUL, 0x8000000000008089UL, 0x8000000000008003UL, 0x8000000000008002UL, 0x8000000000000080UL, 0x000000000000800aUL, 0x800000008000000aUL, 0x8000000080008081UL, 0x8000000000008080UL, 0x0000000080000001UL, 0x8000000080008008UL }; static const uint32_t keccakf_rotc[KECCAK_ROUNDS] = { 1, 3, 6, 10, 15, 21, 28, 36, 45, 55, 2, 14, 27, 41, 56, 8, 25, 43, 62, 18, 39, 61, 20, 44 }; static const uint32_t keccakf_piln[KECCAK_ROUNDS] = { 10, 7, 11, 17, 18, 3, 5, 16, 8, 21, 24, 4, 15, 23, 19, 13, 12, 2, 20, 14, 22, 9, 6, 1 }; void keccak_block_permutation(uint64_t s[SHA3_KECCAK_SPONGE_WORDS]) { int i, j, round; uint64_t t, bc[5]; for (round = 0; round < KECCAK_ROUNDS; round++) { for (i = 0; i < 5; i++) { bc[i] = s[i] ^ s[i + 5] ^ s[i + 10] ^ s[i + 15] ^ s[i + 20]; } for (i = 0; i < 5; i++) { t = bc[(i + 4) % 5] ^ SHA3_ROTL64(bc[(i + 1) % 5], 1); for (j = 0; j < SHA3_KECCAK_SPONGE_WORDS; j += 5) { s[j + i] ^= t; } } t = s[1]; for (i = 0; i < KECCAK_ROUNDS; i++) { j = keccakf_piln[i]; bc[0] = s[j]; s[j] = SHA3_ROTL64(t, keccakf_rotc[i]); t = bc[0]; } for (j = 0; j < SHA3_KECCAK_SPONGE_WORDS; j += 5) { for (i = 0; i < 5; i++) { bc[i] = s[j + i]; } for (i = 0; i < 5; i++) { s[j + i] ^= (~bc[(i + 1) % 5]) & bc[(i + 2) % 5]; } } s[0] ^= keccakf_rndc[round]; } } void sha3_init(void *priv) { struct sha3 *ctx = (struct sha3 *) priv; memset(ctx, 0, sizeof(*ctx)); ctx->capacity_words = (2 * SHA3_HASH_SIZE) / sizeof(uint64_t); } enum sha3_flags sha3_set_flags(void *priv, enum sha3_flags flags) { struct sha3 *ctx = (struct sha3 *) priv; flags &= SHA3_FLAGS_KECCAK; ctx->capacity_words |= (flags == SHA3_FLAGS_KECCAK ? SHA3_USE_KECCAK_FLAG : 0); return flags; } void sha3_update(void *priv, void const *bufIn, size_t len) { struct sha3 *ctx = (struct sha3 *) priv; uint32_t old_tail = (8 - ctx->byte_index) & 0x7; size_t words; uint32_t tail; size_t i; const uint8_t *buf = bufIn; uint64_t t; if (len < old_tail) { while (len--) { ctx->saved |= (uint64_t)(*(buf++)) << ((ctx->byte_index++) * 8); } return; } if (old_tail) { len -= old_tail; while (old_tail--) { ctx->saved |= (uint64_t)(*(buf++)) << ((ctx->byte_index++) * 8); } ctx->u.s[ctx->word_index] ^= ctx->saved; ctx->byte_index = 0; ctx->saved = 0; if (++ctx->word_index == (SHA3_KECCAK_SPONGE_WORDS - SHA3_CW(ctx->capacity_words))) { keccak_block_permutation(ctx->u.s); ctx->word_index = 0; } } words = len / sizeof(uint64_t); tail = len - words * sizeof(uint64_t); for (i = 0; i < words; i++, buf += sizeof(uint64_t)) { t = (uint64_t)(buf[0]) | ((uint64_t)(buf[1]) << 8) | ((uint64_t)(buf[2]) << 16) | ((uint64_t)(buf[3]) << 24) | ((uint64_t)(buf[4]) << 32) | ((uint64_t)(buf[5]) << 40) | ((uint64_t)(buf[6]) << 48) | ((uint64_t)(buf[7]) << 56); ctx->u.s[ctx->word_index] ^= t; if (++ctx->word_index == SHA3_KECCAK_SPONGE_WORDS - SHA3_CW( ctx->capacity_words)) { keccak_block_permutation(ctx->u.s); ctx->word_index = 0; } } while (tail-- > 0) { ctx->saved |= (uint64_t)(*(buf++)) << ((ctx->byte_index++) * 8); } } void const *sha3_exit(void *priv) { struct sha3 *ctx = (struct sha3 *) priv; uint64_t t; uint32_t t1, t2; int i; if (ctx->capacity_words & SHA3_USE_KECCAK_FLAG) { t = (uint64_t)(((uint64_t) 0x01) << (ctx->byte_index * 8)); } else { t = (uint64_t)(((uint64_t)(0x02 | (1 << 2))) << ((ctx->byte_index) * 8)); } ctx->u.s[ctx->word_index] ^= ctx->saved ^ t; ctx->u.s[SHA3_KECCAK_SPONGE_WORDS - SHA3_CW(ctx->capacity_words) - 1] ^= 0x8000000000000000UL; keccak_block_permutation(ctx->u.s); for (i = 0; i < SHA3_KECCAK_SPONGE_WORDS; i++) { t1 = (uint32_t) ctx->u.s[i]; t2 = (uint32_t)((ctx->u.s[i] >> 16) >> 16); ctx->u.sb[i * 8 + 0] = (uint8_t)(t1); ctx->u.sb[i * 8 + 1] = (uint8_t)(t1 >> 8); ctx->u.sb[i * 8 + 2] = (uint8_t)(t1 >> 16); ctx->u.sb[i * 8 + 3] = (uint8_t)(t1 >> 24); ctx->u.sb[i * 8 + 4] = (uint8_t)(t2); ctx->u.sb[i * 8 + 5] = (uint8_t)(t2 >> 8); ctx->u.sb[i * 8 + 6] = (uint8_t)(t2 >> 16); ctx->u.sb[i * 8 + 7] = (uint8_t)(t2 >> 24); } return ctx->u.sb; } void hash_to_string(char string[], const uint8_t hash[SHA3_HASH_SIZE]) { size_t i; for (i = 0; i < SHA3_HASH_SIZE; i++) { string += sprintf(string, "%02x", hash[i]); } } void do_sha3(enum sha3_flags flags, const void *in, uint32_t inBytes, void *out) { struct sha3 c; const void *h; uint8_t hash[SHA3_HASH_SIZE]; sha3_init(&c); if (sha3_set_flags(&c, flags) != flags) { fprintf(stderr, "Error: sha3_set_flags()\n"); exit(1); } sha3_update(&c, in, inBytes); h = sha3_exit(&c); memcpy(hash, h, SHA3_HASH_SIZE); hash_to_string(out, hash); } int main(void) { char buf[BUFSIZE], hash_string[BUFSIZE]; size_t len; printf("Please input a string: "); fgets(buf, BUFSIZE, stdin); len = strlen(buf) - 1; buf[len] = '\0'; printf("buf = %s\n", buf); strcpy(hash_string, buf); do_sha3(SHA3_FLAGS_NONE, hash_string, len, hash_string); printf("SHA3-256: %s\n", hash_string); strcpy(hash_string, buf); do_sha3(SHA3_FLAGS_KECCAK, hash_string, len, hash_string); printf("Keccak-256: %s\n", hash_string); return 0; } |
空の文字列を入力した場合の実行結果は以下になります.
1 2 3 4 5 6 |
$ gcc sha3_keccak.c $ a.out Please input a string: buf = SHA3-256: a7ffc6f8bf1ed76651c14756a061d662f580ff4de43b49fa82d80a4b80f8434a Keccak-256: c5d2460186f7233c927e7db2dcc703c0e500b653ca82273b7bfad8045d85a470 |
以下のリンク先にあるツールで空の文字列を入力して暗号学的ハッシュ関数を実行すると同様の結果になることがわかります.
- SHA3-256:a7ffc6f8bf1ed76651c14756a061d662f580ff4de43b49fa82d80a4b80f8434a
- Keccak-256:c5d2460186f7233c927e7db2dcc703c0e500b653ca82273b7bfad8045d85a470
まとめ
C言語でビットコインとイーサリアムで利用する暗号学的ハッシュ関数を紹介しました.
ビットコインはSHA-2,イーサリアムはKeccakを利用していることがわかりました.
他の代表的な暗号学的ハッシュ関数としてBLAKEがありますので,興味があるあなたは以下のWebサイトで学びましょう!
2022年10月現在,BLAKE3(BLAKEのバージョン3)が最新版です.
C言語を独学で習得することは難しいです.
私にC言語の無料相談をしたいあなたは,公式LINE「ChishiroのC言語」の友だち追加をお願い致します.
私のキャパシティもあり,一定数に達したら終了しますので,今すぐ追加しましょう!
独学が難しいあなたは,元東大教員がおすすめするC言語を学べるオンラインプログラミングスクール5社で自分に合うスクールを見つけましょう.後悔はさせません!